python class decorator wraps
I know there is not very common in Python to use the singleton pattern but I found a nice implementation of this pattern in Python 3 Patterns Recipes and Idioms book. Decoration is a way to specify management code for functions and classes.
Mastering Decorators In Python 3 Ever Wondered How A Python Decoration By Arian Seyedi Level Up Coding
A Python decorator wraps a target function with another wrapper function.
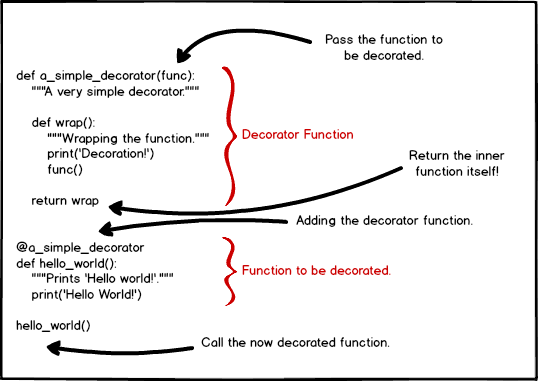
. Is an adapter see the Adapter Pattern. Do something with arg1 and arg2 and then pass the modified values to the wrapped function. Python decorator get class name.
Decorators themselves take the form of callable objects that process other callable objects. Decorators are an important feature in Python. Questions numpy 318 Questions opencv 66 Questions pandas 1025 Questions pip 64 Questions pygame 63 Questions python 6023 Questions python-27 67 Questions python-3x 688 Questions regex 105 Questions.
They are also known as decorators. Python Decorators Decorators Decorators are a structural design pattern which wrap the original object to add extra functionality dynamically without modifying the original object. Raise TypeErrorMethod s forces keyword arguments.
A few of them are. Functoolswraps cls updated class D cls. That delegates method calls to the object it wraps.
Do something before the function call result wrapped_function args kwargs do something after the function call return result return _wrapper decorator with functoolswraps added def. Contextmanager def wrapping_logic. May be you can find better way to do it but for example you can just move your wrapping logic to some context manager to prevent code duplication.
Decorators can be thought of as a function wrapper meaning that it takes in a function as an argument and return a modified version of the function adding extensions or capabilities to it. Import functools def decoratorfunc. Import functools def dec cls.
Decorators are usually used to run code before andor after a function. Import asyncio import functools import time from contextlib import contextmanager def duration func. How to create a Python decorator that can wrap either coroutine or function.
Functions that act on or return other functions. Simple lightweight unbounded function cache. Structure of a Decorator.
Now create the database connection class. The decorator classs purpose is to add to remove from or adjust the behaviors that the wrapped object would normally implement when its methods are called. In general any callable object can be treated as a function for the purposes of this module.
Decorators are a very powerful and useful tool in Python since it allows programmers to modify the behaviour of function or class. PrintBefore orginal function with decorator args arg1 arg2 result fargs kwargs printRan after the orginal function return result return wrapper return decorator decorator_func_with_argstest1 test2 def helloname. By May 8 2022 best tres leches cake recipe ffpc main event winners May 8 2022 best tres leches cake recipe ffpc main event winners.
Def _executearg1 arg2 _args _kwargs. In writing a class decorator of this type __call__ should return an ordinary. Use a Base Class.
A Python module for decorators wrappers and monkey patching Aug 23 2021 3 min read wrapt The aim of the wrapt module is to provide a transparent object proxy for Python which can be used as the basis for the construction of function wrappers and decorator functions. Decorators are a highly effective and helpful Python feature because we can use them to change the behavior of a function or class. Functools is a standard Python module for higher-order functions functions that act on or return other functions.
To limit concurrent access to a shared resource. The functools module defines the following functions. This can be done using a nested function which in turn then calls the wrapped function.
The decorator function name has the symbol as its prefix to. Turns out theres a straightforward solution using functoolswraps itself. Decorated 1 return D dec class C.
From functools import wraps def decorator_func_with_argsarg1 arg2. PrintHello say_hello decoratorsay_hello say_hello But using the syntax it can be simplified to this. Doc print f C__name__ C__doc__ C__wrapped__.
We use them to wrap another function in order to enhance its functionality without having to change it permanently. In Python the most common syntax to use decorators is to place the decorator function above the to-be-decorated function. Wrapper Class in Python.
In Python decorators can be either functions. What are Wrappers in Python. So wrappers are the functionality available in Python to wrap a function with another function to extend its behavior.
Func printGoodbye return say_goodbye def say_hello. Wraps is a decorator that is applied to the wrapper function of a decorator. Now the reason to use wrappers in our code lies in the fact that we can modify a wrapped function without actually changing it.
The wrapt module focuses very much on correctness. Def keyword_onlyfunc self args kwargs. Wraptdecorator def my_decoratorwrapped instance args kwargs.
A decorator that forces keyword arguments in the wrapped method and saves actual input keyword arguments in _input_kwargs. Using a decorator we can define a class as a singleton by forcing the class to either return an existing instance of the class or create. Import functools Part of Python standard library def decoratorwrapped_function.
That implements the same interface as the object it wraps. The functools module is for higher-order functions. This pattern creates a decorator class which wraps the original class and provides.
For example it can track execution times redefine how the wrapped function runs or modify return values. This wrapper function can add any behavior you might want. This wrapper adds some additional functionality to existing code.
A Class Decorator is similar to function decorators but they are run at the end of a class statement to rebind a class name to a. Wrapsf def wrapperargs kwargs. At the moment our code looks like this.
These are decorators that do not accept arguments. In Python the most common syntax to use decorators is to place the decorator function above the to-be-decorated function. In essence decorators are functions that modify the behavior of decorated functions without changing their innate functionalities.
When we decorate a function with a class that function becomes an instance of the class. Singleton decorator python. Should only be used to wrap a method where first arg is self if lenargs 0.
As a concrete example the standard librarys functoolslru_cache decorator wraps a target function to add caching. How to wrap a decorator around another classes method. It updates the wrapper function to look like wrapped function by copying attributes such as __name__ __doc__ the docstring etc.
Handling Exceptions In Python A Cleaner Way Using Decorators By Shivam Batra The Startup Medium
Infographic Note On Introduction To Machine Learning Using Pytorch With Sample Questions And Ans Introduction To Machine Learning Data Science Machine Learning
Python Decorators With Examples Python Python Programming Geek Stuff
What You Probably Don T Know About Python Decorators Hackernoon
How To Use Python Property Decorator Askpython
Decorators In Python Step By Step Guide To Python Decorators By Probhakar Medium
Python Decorators Guide Mybluelinux Com
How Python Decorators Work 7 Things You Must Know Codefather
Quick Tutorial Introduction To Python Decorators
Try Catch General Decorator To Wrap Try Except In Python Stack Overflow
Python Decorators In A Nutshell Dev Community
Python Decorators Explained If You Ve Spent Even A Little Bit Of By Matt Harzewski The Startup Medium
Advanced Uses Of Python Decorators Codementor
Class Based Decorators Make Your Python Code Classy Idego Group
Decorator In Python How To Use Decorators In Python With Examples
Class Based Decorators Make Your Python Code Classy Idego Group
Decorated Plugins Kaleidoescape Linguist Turned Programmer
Python Decorator With Arguments Python Decorator Tutorial With Example Dev Community
Decorators In Python Step By Step Guide To Python Decorators By Probhakar Medium